Python is a powerful yet easy-to-learn general-purpose programming language available under the GNU General Public License (GPL). Python is the most important language you can learn if you want to be a successful IT professional.
In any job, the first step is to prepare well for the interview. Here are some advanced questions asked in python interviews and answers for experienced candidates to help you breeze through your following Python interview after completing a python course.
1. In Python, how does memory management work?
The Python Memory Manager is responsible for all aspects of memory management in it. Python has its own private heap space in the manager’s memory allocation. This private heap contains all Python objects, and the programmer cannot access them. The personal heap space is not absent from the Python API, however.
Python’s built-in garbage collection recycles the unused memory in the private heap space.
2. What are Python namespaces? Why are they used?
A namespace in Python ensures that all object names in a program are unique and can be used without conflict. Like dictionaries, these namespaces are mapped to an object with the same name as the key. In this way, multiple namespaces can share a single name and map it to a different thing.
3. In Python, what is the meaning of “Scope Resolution”?
Objects with the same name but different functions are not uncommon. Python automatically handles scope resolution in these cases. Some examples of this include:
Many functions in the Python modules ‘math’ and ‘cmath’ are shared between them, such as log10(), acos(), and exp(). Prefixing them with their respective modules, such as math.exp() and cmath.exp, is necessary to clear any confusion ().
A temp object has been initialized to 10 globally and then to 20 on each function call in the following code. The call to the function, however, did not affect the temp’s global value. There is a clear distinction between global and local variables, indicating that it treats their namespaces as distinct entities.
4. In Python, what are “decorators”?
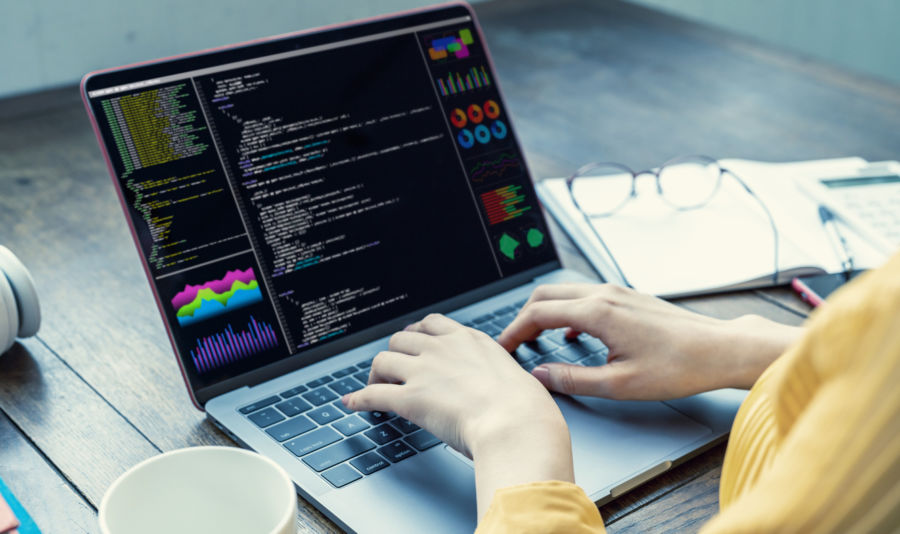
Functions that add additional functionality to an existing function in Python without altering the structure of the function are known as decorators. In Python, the @decorator name represents them, and they are accessed from the bottom up.
Because of their ability to accept arguments for functions and further modify them before passing them on to the function itself, decorators are an excellent addition to any programming language. The ‘wrapper’ function, which is an inner nested function, is critical in this case. It is designed to keep itself hidden from the rest of the program’s scope by enforcing encapsulation. You can get to learn more about these during the python course.
5. What is the difference between a Dict and a List?
Comprehended lists, dictionaries, and sets can be built from a given list, dictionary, or set with the help of Python comprehensions, which are similar to decorators. Confusions save a lot of time and code that would otherwise be a lot longer if they weren’t used (containing more lines of code).
Also read: How to Become An Ethical Hacker and Get Your Dream Job
6. In Python, how do you copy an object?
No objects are copied in Python when you use the = operator (assignment statement). Because the target variable name already exists, it creates a binding. It’s copy module is used to make a copy of an object. To make additional copies of a given object, you can use the copy module in one of two ways:
A Shallow Copy is known as a bit-by-bit copy of the original object. The values of the copied object are identical to those of the original object. Any references to other objects in either of the deals are merely copied, not the actual objects themselves.
Deep Copy duplicates all objects referenced by the source object, copying all values from the source to the target.
7. How range and xrange in Python are different?
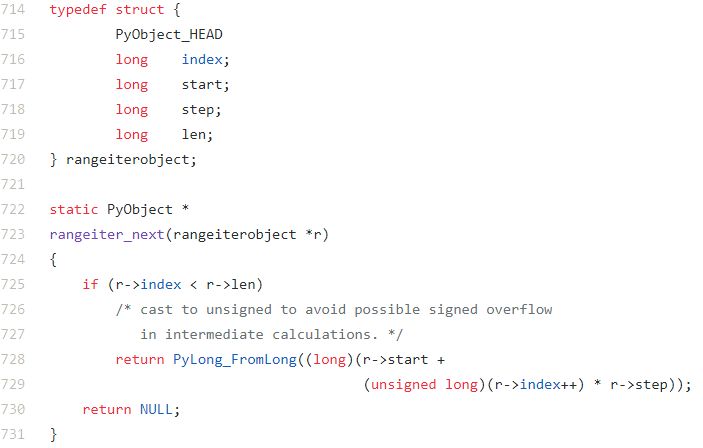
In terms of functionality, xrange() and range() are very similar. xrange() returns an xrange object, whereas the range() function returns a Python list. This is the only difference.
What difference does that make? Since xrange() doesn’t generate a static list like range(), it creates the value on the fly instead. The term “yielding” has been coined to describe this approach when used with an object-type generator.
In memory-constrained applications, yielding is a must. A Memory Error can occur if a static list is created as in range(), whereas xrange() uses just enough memory for the generator (significantly less in comparison). You can learn much more with a few examples and practical teaching during the python course.
8. What are pickling and unpickling?
Serialization is a built-in feature of the Python library. Serialization is the process of transforming an object into a format to be stored. It can later be able to deserialized for getting back to the original object. When it comes to this, the pickle module comes in handy.
Pickling
Python’s serialization process is referred to as “pickling.” A byte stream of any Python object can be dumped into memory as a file. Even though pickling is a relatively simple process, pickle products can be compressed even more. Additionally, pickle keeps track of the serialized objects it has, and the serialization is reusable across different versions of the software. The pickle.dump function is used for this task ().
Unpickling
It is the opposite of pickling. When the byte stream is deserialized, it loads the file’s stored objects into memory.
Pickle is the function that is used in the above procedure.
load().
9. What are generators in Python?
An iterable collection of items is returned by a generator function one at a time in a predefined manner. Iterators can be created using generators in a variety of ways. Instead of returning a generator object, they use the yield keyword.
10. What is PYTHONPATH?
It is possible to add additional directories to the PYTHONPATH environment variable that it will search for modules and packages. Maintaining Python libraries that are not installed in the global default location is made easier with this feature.
These are some advanced questions and answers that can be quickly answered when you get a python course.
Also read: How to Become a Full Stack Developer: Tips